Swift5.1.3 Xcode11.3.1 SwiftUI
- How to create digital d6 dice on your app
- Change the random rate for tricks of game
- Import GameplayKit with SwiftUI for easy development
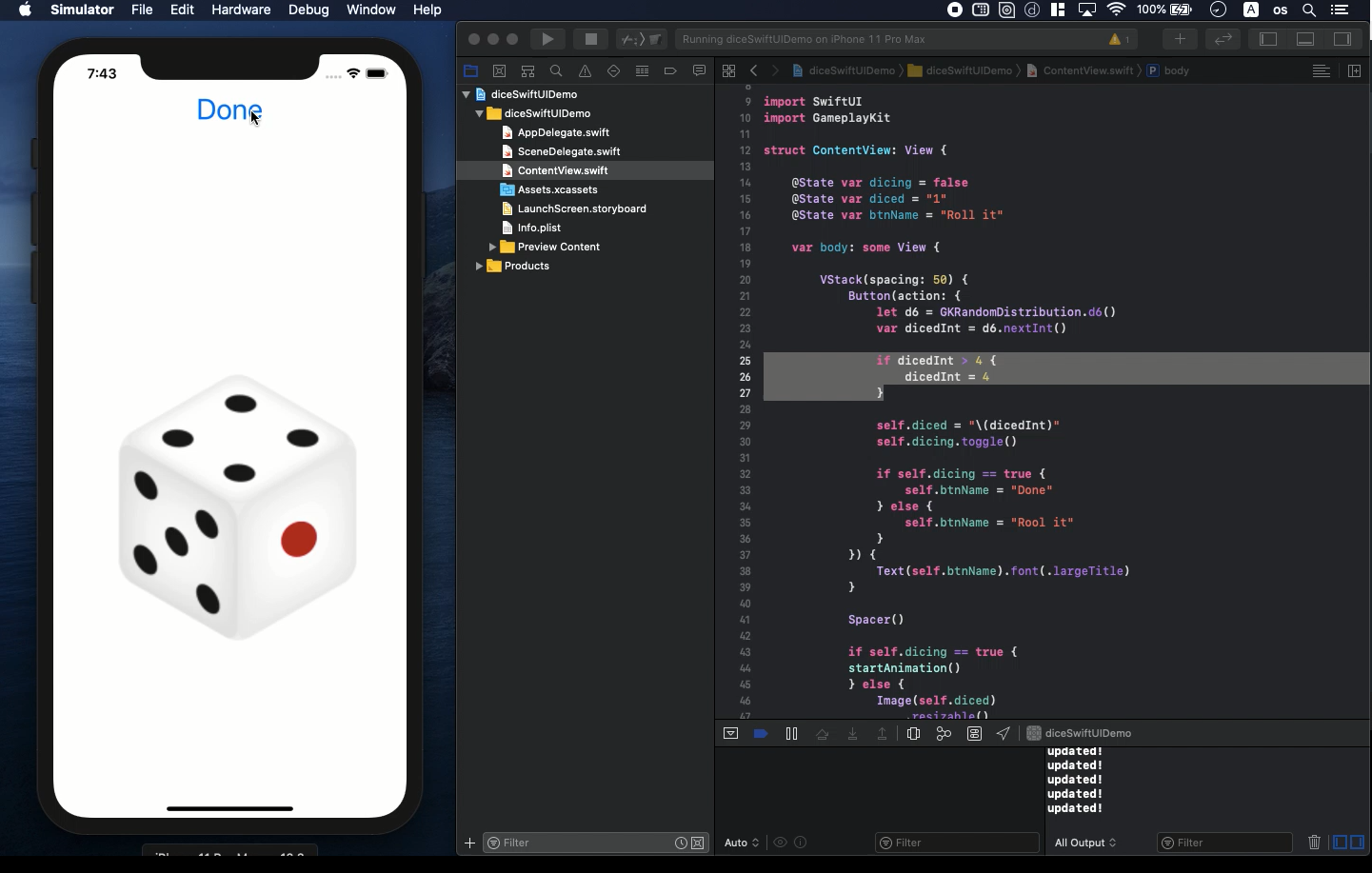
* Here is the highlight of YouTube tutorial.
By using GameplayKit library, you can easily create digital d6 dice roller
with SwiftUI. Let me show you how the standard virtual dice works in your
app at first.
Please prepare some dice pictures from 1 to 6 and install them into Assets.xcassets
of the project. The file names are as follows;
1.png
2.png
3.png
4.png
5.png
6.png
To make the animation of rolling dice, you need the following struct.
var images : [UIImage]! = [UIImage(named: "1")!, UIImage(named:
"4")!, UIImage(named: "2")!, UIImage(named: "3")!,
UIImage(named: "5")!, UIImage(named: "6")!]
let animationImage = UIImage.animatedImage(with: images, duration: 2.0)
struct startAnimation: UIViewRepresentable {
func makeUIView(context: Self.Context) -> UIView {
let myView = UIView(frame: CGRect(x: 0, y: 0, width: 400, height: 400))
let myImage = UIImageView(frame: CGRect(x: 30, y: 200, width: 360, height:
300))
myImage.contentMode = UIView.ContentMode.scaleAspectFill
myImage.image = animationImage
myView.addSubview(myImage)
return myView
}
func updateUIView(_ uiView: UIView, context: UIViewRepresentableContext<startAnimation>)
{
print("updated!")
}
}
The flash images of these 6 pictures appears rolling dice. The point of
this appearance is the following array.
var images : [UIImage]! = [UIImage(named: "1")!, UIImage(named:
"4")!, UIImage(named: "2")!, UIImage(named: "3")!,
UIImage(named: "5")!, UIImage(named: "6")!]
You should not place the pictures in order. And, the center of these pictures
should be shifted a little. In this way, the virtual dice appears to move
actually.
Let's check the movement by using startAnimation() in ContentView.
Next, you need to hack the function of dice rate programatically.
But, don't worry it is so simple. After you import the library, only two
lines are what you should add.
import GameplayKit
let d6 = GKRandomDistribution.d6()
var dicedInt = d6.nextInt()
The number (Int) of 1 to 6 will be assigned randomly to the variable, dicedInt.
This function works as the roller of dice.
With the active timing of this function, convert the fixed picture to the
animation like this.
if self.dicing == true {
startAnimation()
} else {
Image(self.diced)
.resizable()
.aspectRatio(contentMode: .fit)
}
Let me summerize the code.
@State var dicing = false
@State var diced = "1"
@State var btnName = "Roll it"
var body: some View {
VStack(spacing: 50) {
Button(action: {
let d6 = GKRandomDistribution.d6()
var dicedInt = d6.nextInt()
// if dicedInt > 4 {
// dicedInt = 4
// }
self.diced = "\(dicedInt)"
self.dicing.toggle()
if self.dicing == true {
self.btnName = "Done"
} else {
self.btnName = "Rool it"
}
}) {
Text(self.btnName).font(.largeTitle)
}
Spacer()
if self.dicing == true {
startAnimation()
} else {
Image(self.diced)
.resizable()
.aspectRatio(contentMode: .fit)
}
}
}
In this code above, you can create the virtual d6 dice in your app.
You may find some part is commented out. You know why?
Yes, it is the cheat code.
In this code, 5 and 6 will be converted to 4 every time.
So, if you uncomment it, the random rate will be changed.
- 5 and 6 will not appear.
- 4 will appear by 50%.
- 1, 2 and 3 will appear in the same rate for each other.
If you know this rate in some games, I believe you would never lose. You
can change the code as you want.
To get the source code, check the comment of my YouTube
Back to Table List